This sample shows three variations how to add a watermark to an
existing PDF file.
See the PDF file created by this sample: Watermark.pdf (238 kB) Note: this sample requires Acrobat Reader 5.0 or higher because it uses / demonstrates transparency
Variation 1 - Draw watermark as text string (click image to enlarge):
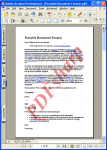 | Draw watermark as text string |
// Variation 1: Draw watermark as text string
// Get an XGraphics object for drawing beneath the existing content
XGraphics gfx = XGraphics.FromPdfPage(page, XGraphicsPdfPageOptions.Prepend);
// Get the size (in point) of the text
XSize size = gfx.MeasureString(watermark, font);
// Define a rotation transformation at the center of the page
gfx.TranslateTransform(page.Width / 2, page.Height / 2);
gfx.RotateTransform(-Math.Atan(page.Height / page.Width) * 180 / Math.PI);
gfx.TranslateTransform(-page.Width / 2, -page.Height / 2);
// Create a string format
XStringFormat format = new XStringFormat();
format.Alignment = XStringAlignment.Near;
format.LineAlignment = XLineAlignment.Near;
// Create a dimmed red brush
XBrush brush = new XSolidBrush(XColor.FromArgb(128, 255, 0, 0));
// Draw the string
gfx.DrawString(watermark, font, brush,
new XPoint((page.Width - size.Width) / 2, (page.Height - size.Height) / 2),
format);
Variation 2 - Draw watermark as outlined graphical path (click image to enlarge):
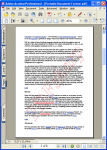 | Draw watermark as outlined graphical path |
// Variation 2: Draw watermark as outlined graphical path
// Get an XGraphics object for drawing beneath the existing content
XGraphics gfx = XGraphics.FromPdfPage(page, XGraphicsPdfPageOptions.Prepend);
// Get the size (in point) of the text
XSize size = gfx.MeasureString(watermark, font);
// Define a rotation transformation at the center of the page
gfx.TranslateTransform(page.Width / 2, page.Height / 2);
gfx.RotateTransform(-Math.Atan(page.Height / page.Width) * 180 / Math.PI);
gfx.TranslateTransform(-page.Width / 2, -page.Height / 2);
// Create a graphical path
XGraphicsPath path = new XGraphicsPath();
// Add the text to the path
path.AddString(watermark, font.FontFamily, XFontStyle.BoldItalic, 150,
new XPoint((page.Width - size.Width) / 2, (page.Height - size.Height) / 2),
XStringFormat.Default);
// Create a dimmed red pen
XPen pen = new XPen(XColor.FromArgb(128, 255, 0, 0), 2);
// Stroke the outline of the path
gfx.DrawPath(pen, path);
Variation 3 - Draw watermark as transparent graphical path above text (click image to enlarge):
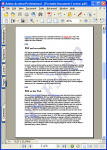 | Draw watermark as transparent graphical path above text |
// Variation 3: Draw watermark as transparent graphical path above text
// Get an XGraphics object for drawing above the existing content
XGraphics gfx = XGraphics.FromPdfPage(page, XGraphicsPdfPageOptions.Append);
// Get the size (in point) of the text
XSize size = gfx.MeasureString(watermark, font);
// Define a rotation transformation at the center of the page
gfx.TranslateTransform(page.Width / 2, page.Height / 2);
gfx.RotateTransform(-Math.Atan(page.Height / page.Width) * 180 / Math.PI);
gfx.TranslateTransform(-page.Width / 2, -page.Height / 2);
// Create a graphical path
XGraphicsPath path = new XGraphicsPath();
// Add the text to the path
path.AddString(watermark, font.FontFamily, XFontStyle.BoldItalic, 150,
new XPoint((page.Width - size.Width) / 2, (page.Height - size.Height) / 2),
XStringFormat.Default);
// Create a dimmed red pen and brush
XPen pen = new XPen(XColor.FromArgb(50, 75, 0, 130), 3);
XBrush brush = new XSolidBrush(XColor.FromArgb(50, 106, 90, 205));
// Stroke the outline of the path
gfx.DrawPath(pen, brush, path);
|